POST /users/assignment
Request parameters
Parameter | Type | Description |
---|---|---|
relId | long | Assignment ID relId property from organizations[] object |
role | string | Possible roles: "employee", "approver", "manager", "administrator", "main_administrator" role property in roles[] users object |
userId | string | Provide the user id for whom the assignment should be added or edited. |
organizationId | string | Provide the organization id for which organization the assignment should be added or edited. id property in organizations[] object |
validFrom | date | Date from when the assignment becomes valid. Provide date in ISO-8601 and UTC format with +00:00 time zone, eg. 2022-01-01T00:00:00+00:00 If left empty, then default date 1970-01- 01T00:00:00+00:00 will be saved. validFrom property in organizations[] object |
validTo | date | Date to until the assignment is valid and until user can selected the organization for time entries. Provide date in ISO-8601 and UTC format with +00:00 time zone, eg. 2022-01-01T00:00:00+00:00 If left empty, then default date 2200-01-01T00:00:00+00:00 will be saved. validFrom property in organizations[] object |
Request JSON example
{
"relId": 3520278,
"role": "approver",
"userId": "7564942a-fb06-4f81-ba2f-9d8ee849d2a1",
"organizationId": "2491a1a2-3470-4e18-808b-c1e2a9abb8a6",
"validFrom": "1970-01-02T00:00:00+00:00",
"validTo": "2023-12-31T00:00:00+00:00"
}
Description of mapping "roles" and "organizations" from GET /users/{userId} arrays to the update assignment request body
{
"role": "approver", // => role
"organizations": [
{
"id": "2491a1a2-3470-4e18-808b-c1e2a9abb8a6", // => organizationId
"name": "Sub-task 1.1",
"relId": 3520278, // => relId
"validFrom": "1970-01-01T00:00:00+00:00", // => validFrom
"validTo": "2023-12-31T00:00:00+00:00" // => validTo
}
]
},
Success Reponses
Code | Title | Description |
---|---|---|
200 | Success | Returned if request was successful. |
400 | Bad Request | Returned if request was bad or any other error occured. |
401 | Unauthorized | Returned if request was not authorized (eg. due to bad API key). |
404 | Not Found | Returned if request could not be found. |
Users permissions will be considered
The response will consider the permissions of the user who is authenticated by the API call and will only allow assignments to organizations which user is allowed perform.
Success respone JSON example for successfully creating new assignment
{
"items": [
{
"message": "The assignment was successfully created.",
"data": null
} ],
"valid": null
Success respone JSON example for successfully editing an assigment
{
"items": [
{
"message": "The assignment was successfully updated.",
"data": null
}
],
"valid": null
}
Conflict Reponses
Assignement dates may not overlapp. In case a request is sent where assignment dates are overlapping, the api will return a conflictType response with a suggestion how to solve the conflict.
This suggestion can be followed or the conflict can be resolved, as needed, in another way.
Parameter | Description |
---|---|
relId | Assignment ID |
roles | Array of assignment roles Example: role: [“employee”] role: [“administrator”, “approver”, “employee”] |
userId | Id of user |
organizationId | Id of assignment organization |
conflictType | Type of conflict. Shows the suggestion how the conflicts can be resolved.no_update – no updates are required for the assignmentdate_from_updated – validFrom date should be updated to resolve the conflictdate_to_updated – validTo date should be updated to resolve the conflictto_delete – assignments should be completely deleted to resolve the conflict |
validFrom | Suggested assignment valid from date (ISO date). |
validTo | Suggested assignment valid to date (ISO date). |
validated | Resolving status.false – requires resolving.true – conflict is resolved. |
Conflict response JSON example where new create request overlaps with relId = 3455916
{
"items": [
{
"message": "Dates may not overlap. Api, User has already a role in the organization unit Test 3.1.2 which is in conflict with the new permission.",
"data": [
{
"relId": 3455916,
"userId": "0e90fa19-f60a-4ff9-960a-6c56747d19d5",
"organizationId": "dc71dd83-356a-4e5b-8510-32a844c3d825",
"roles": [
"employee",
"administrator"
],
"validFrom": "1970-01-01T00:00:00+00:00",
"validTo": "2020-12-31T00:00:00+00:00",
"conflictType": "date_to_updated",
"validated": false
},
{
"relId": null,
"userId": "0e90fa19-f60a-4ff9-960a-6c56747d19d5",
"organizationId": "dc71dd83-356a-4e5b-8510-32a844c3d825",
"roles": [
"employee"
],
"validFrom": "2021-01-01T00:00:00+00:00",
"validTo": "2200-01-01T00:00:00+00:00",
"conflictType": "no_update",
"validated": true
}
]
}
],
"valid": null
}
Conflict response JSON example where new create request has conflictType : "date_to_updated"
{
"items": [
{
"message": "Dates may not overlap. TestApi, User has already a role in the organization unit Sub-task 1.2 which is in conflict with the new permission.",
"data": [
// AssignmentConflictItem
{
// exiting assignment
"relId": 2507281,
"userId": "7564942a-fb06-4f81-ba2f-9d8ee849d2a1",
"groupId": "9bf007ac-243e-44db-828d-1fb11fa49262",
"roles": [
"employee"
],
"validFrom": "1970-01-01T00:00:00+00:00",
"validTo": "2020-12-31T00:00:00+00:00",
"conflictType": "date_to_updated",
"validated": false
},
{
// new assignment
"relId": null,
"userId": "7564942a-fb06-4f81-ba2f-9d8ee849d2a1",
"groupId": "9bf007ac-243e-44db-828d-1fb11fa49262",
"roles": [
"employee"
],
"validFrom": "2021-01-01T00:00:00+00:00",
"validTo": "2200-01-01T00:00:00+00:00",
"conflictType": "no_update",
"validated": true
}
]
}
],
"valid": null
}
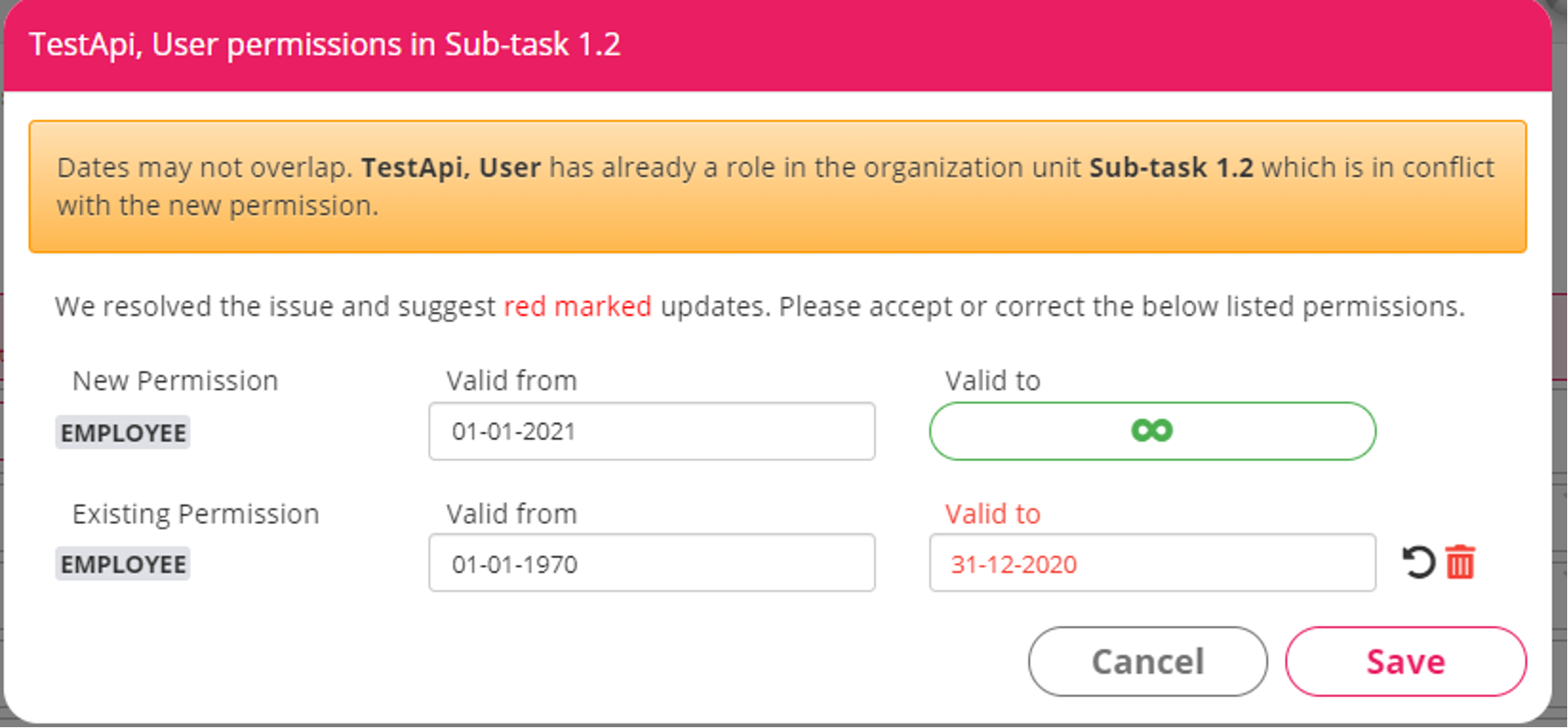
Example of the same assignment conflict in Web App